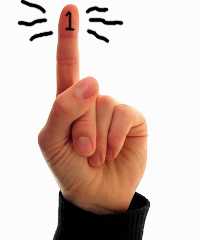
class ProgramConfiguration{ public ProgramConfiguraiton(){ //default constructor code } }A new instance of a class is created by the constructor. Most of the time, we have a public constructor, which is called to create a new instance. Since we want to prohibit multiple instance, we have to restrict access to the constructor. This is done by making the constructor private.
class ProgramConfiguration{ private ProgramConfiguration(){ //default private constructor code } }then we create a static public method that will make sure that only one instance lives in the whole program.
class ProgramConfiguration{ private static ProgramConfiguration _configObject; private ProgramConfiguration(){ //default private constructor code } public getInstance(){ /* if an instance exist return that instance otherwise call the constructor to create an instance and return it. */ if(_configObject == null){ _configObject = ProgramConfiguration(); } return _configObject; } }So anytime you want to get that single object, you call the getInstance() method.
public static void main(String[] args){ /* no access to default constructor. so if you did ProgramConfiguration pc = new ProgramConfiguration(); you will get compilation error. */ ProgramConfiguration pc = ProgramConfiguration.getInstance(); ProgramConfiguration newpc = ProgramConfiguration.getInstance(); /* in the above code pc and newpc both point to the same static object. when getinstance() is called for the second time, it finds that _configObject is not null anymore, so it doesn't call the constructor to create any new instance. */ }
I’m not sure which language you’ve used to describe the example. Is it C++ or Java or…? You’ve used “new” and “main()” in the same example.
Hello Peter, I am using java.
I will fix the main() to
public static void main(String args[]){}
thanks for catching that.