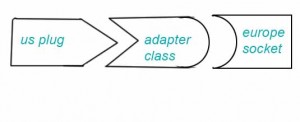
public class USPlug { public USPlug(){ System.out.println("I am a US plug"); } public void plugIn(){ System.out.println("I fit into a square socket"); } }And then there is Europe Plug
public class EuropePlug { public EuropePlug(){ System.out.println("I am a Europe Plug"); } public void plugIn(){ System.out.println("I fit into circle socket"); } }We need to put a US plug into a Europe socket. So we need an adapter called UStoEuropeAdapter. This adapter extends the feature of US plug to be compatible with european socket. So here is the adapter
public class UStoEuropeAdapter implements USPluggable { EuropePlug eplug; public UStoEuropeAdapter(EuropePlug ep){ this.eplug = ep; System.out.println("I am the UStoEurope Adapter"); } public void plugIn() { this.eplug.plugIn(); } }The USPluggable interface mandates a class which implements this to make sure to define method to plug into us socket. ie plugIntoSquareSocket()
public interface USPluggable { public void plugIn(); }The caller program creates europe plug, wraps it into the adapter and plugs it into the square socket. But since the adapter overwrites the method plugIntoSquareSocket() by calling the plugIntoCircleSocket() instead, now this adapter plugs into circle socket.
public class MainProgram { public static void main(String[] args) { EuropePlug ep = new EuropePlug(); USPluggable myAdapter = new UStoEuropeAdapter(ep); myAdapter.plugIn(); } }