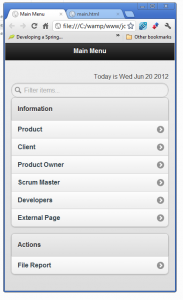
<link rel="stylesheet" href="http://code.jquery.com/mobile/1.0a4.1/jquery.mobile-1.0a4.1.min.css" /> <script type="text/javascript" src="http://code.jquery.com/jquery-1.5.2.min.js"></script> <script type="text/javascript" src="http://code.jquery.com/mobile/1.0a4.1/jquery.mobile-1.0a4.1.min.js"></script>With Jquery Mobile the html tags can have special property called ‘data-role’ which can have different values to create different mobile effects.
tag | data-role |
---|---|
div | page |
div | dialog |
ul/ol | listview |
li | divider |
nav | navbar |
a | button |
a,button | control-group |
field container | fieldcontain |
<div data-role="listview">
<section data-role="page" id="main"> section tag is html5 tag.</section>JQuery mobile works perfectly with html5.
<!DOCTYPE html> <html> <head> <title>Page Title</title> <link rel="stylesheet" href="http://code.jquery.com/mobile/1.0a4.1/jquery.mobile-1.0a4.1.min.css" /> <script type="text/javascript" src="http://code.jquery.com/jquery-1.5.2.min.js"></script> <script type="text/javascript" src="http://code.jquery.com/mobile/1.0a4.1/jquery.mobile-1.0a4.1.min.js"></script> </head> <body> <!--====================================================================--> <section data-role="page" id="main"> <header data-role="header"> <h1>Main Menu</h1> </header> <!--/header --> <div data-role="content"> <p style="text-align:right">Today is <script type="text/javascript"> var d = new Date(); document.write(d.toDateString()); </script></p> <div data-role="listview" data-inset="true" data-filter="true" style="margin-top:10px"> <ul> <li> <h2>Information</h2> </li> <li> <a href="#product">Product</a> </li> <li> <a href="#client">Client</a> </li> <li> <a href="#product_owner">Product Owner</a> </li> <li> <a href="#scrum_master">Scrum Master</a> </li> <li> <a href="#developers">Developers</a> </li> <li> <a href="http://localhost/jquery_mobile_practice/external.html">External Page</a> </li> </ul> </div> <!--listview--> <div data-role="listview" data-inset="true" style="margin-top:10px"> <ul> <li> <h2>Actions</h2> </li> <li> <a href="#form_report">File Report</a> </li> </ul> </div> <!--listview--> </div> <!--/content--> </section> <!-- /page --> <!--================================================================--> <section data-role="page" id="client"> <header data-role="header"> <h1>EZ-IT Solutions</h1> </header> <!--/header --> <div data-role="content"> <h2>Umbrellapia</h2> <p>Umbrellapia has been in the business of making luxury umbrellas since 1990. Their high end umbrellas are sold worldwide. Some of their products include 22k gold handle umbrella, auto open close umbrella and super portable giant umbrella.</p> </div> <!--/content--> </section> <!-- /page --> <!--==================================================================--> <section data-role="page" id="product"> <header data-role="header"> <h1>EZ-IT Solutions</h1> </header> <!--/header --> <div data-role="content"></div> <!--/content--> </section> <!-- /page --> <!--====================================================================--> <div data-role="page" id="product_owner"> <header data-role="header"> <h1>EZ-IT Solutions</h1> </header> <!--/header --> <div data-role="content"></div> <!--/content--> </div> <!-- /page --> <!--=====================================================================--> <section data-role="page" id="scrum_master"> <header data-role="header"> <h1>EZ-IT Solutions</h1> <nav data-role="navbar"> <ul> <li> <a href="#">One</a> </li> <li> <a href="#">Two</a> </li> <li> <a href="#">Three</a> </li> <li> <a href="#">Four</a> </li> </ul> </nav> </header> <!--/header --> <div data-role="content"></div> <!--/content--> </section> <!-- /page --> <!--============================================================--> <section data-role="page" id="form_report"> <header data-role="header"> <h1>EZ-IT Solutions</h1> </header> <!--/header --> <div data-role="content"> <form> <label for="slider-0">Percentage Completed</label> <input type="range" name="slider-0" id="slider-0" value="75" min="0" max="100" /> <a href="#" data-role="button" data-icon="check">Submit</a></form> </div> <!--/content--> </section> <!-- /page --> <!--===========================================================--> <section data-role="page" id="developers"> <header data-role="header"> <h1>EZ-IT Solutions</h1> </header> <!--/header --> <div data-role="content"> <a href="external.html" data-transition="fade">I will fade</a> <br /> <a href="external.html" data-transition="pop">I will pop</a> <br /> <a href="external.html" data-transition="flip">I will flip</a> <br /> <a href="external.html" data-transition="slide">I will slide</a> <br /> <a href="external.html" data-transition="slideup">I will slideup</a> <br /> <a href="external.html" data-transition="slidedown">I will slidedown</a> <br /> <br /> <br /> <a href="external.html" data-transition="fade" data-rel="dialog">I will fade</a> <br /> <a href="external.html" data-transition="pop" data-rel="dialog">I will pop</a> <br /> <a href="external.html" data-transition="flip" data-rel="dialog">I will flip</a> <br /> <a href="external.html" data-transition="slide" data-rel="dialog">I will slide</a> <br /> <a href="external.html" data-transition="slideup" data-rel="dialog">I will slideup</a> <br /> <a href="external.html" data-transition="slidedown" data-rel="dialog">I will slidedown</a> <br /> <a href="#main" data-transition="slidedown" data-rel="popup">I will slidedown</a> <br /> </div> <!--/content--> </section> <!-- /page --> </body> </html>